Using Python to Find Songs with Featured Artists
Okay, today’s edition of 66 Days of Math and Programming is yet again about my Spotify Python project. I can’t help myself. I spend a lot of time on it, so I’ve gotta share the juicy details.
Here’s a block of code I wrote yesterday as part of my scoring algorithm.
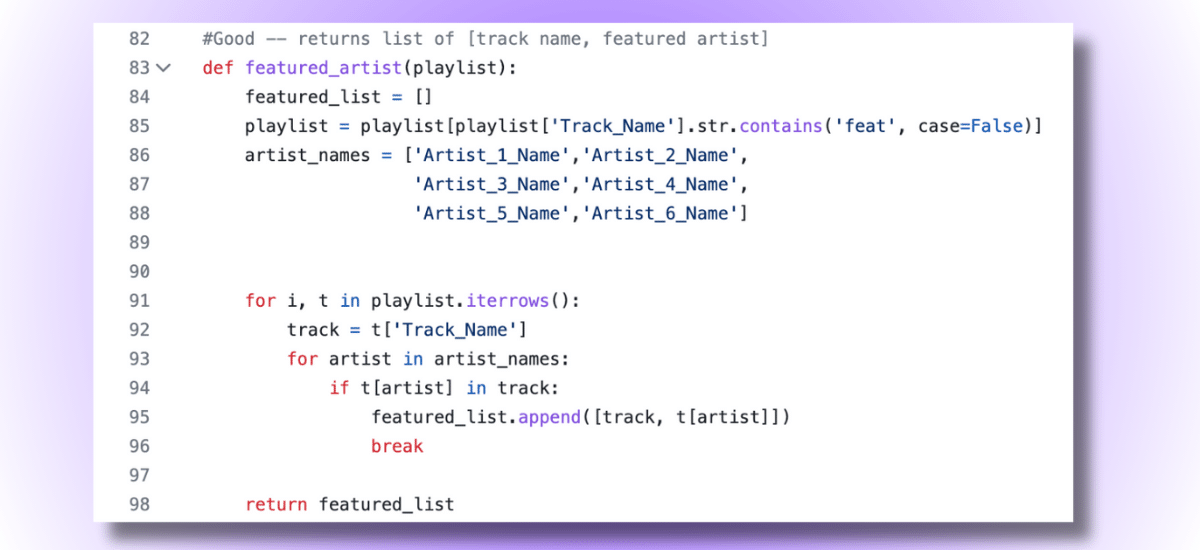
Before diving into the line-by-line, let me explain what this function does. The function takes a playlist parameter that’s a CSV file or table of data containing information about songs, such as Track Name and all Artist Names. It iterates through all track names containing ‘feat’ and returns the songs’ names and the featured artists on the tracks.
Okay, let’s break it down now…
Line 84
I define the empty list that’ll store my return values later on.
Line 85
Here I temporarily redefine the playlist variable from the parameter to only be the rows that contain ‘feat’ (Uppercase or lowercase) in the ‘Track_Name’ column.
Line 86
In this line, I create a list of strings that contains the column names in the playlist table with artist name values.
Line 91
Here’s where the code gets fun. This for loop iterates through every row in the newly defined playlist table.
Line 92
Defining track as the column containing the row’s song name helps me in the next step where…
Line 93
I iterate through every column in the newly defined playlist table using the strings in the artist name list as the index value.
Lines 94-96
As I reiterate through the columns, if an artist name is in the track name (Miedito o Que? (feat. KAROL G)). I add the track name and artist name to the featured_list. And the break statement exists in the for loop so that we don’t keep iterating through the row unnecessarily.
Realizations
This is the beauty of my 66 Days of Math and Programming experiment.
Writing about this code snippet, made me take a deeper look at how my code functions, and I’ve realized three mistakes I made.
- Some songs have more than one featured artist, and the break statement limits the values I save to one per song
- Some song names contain phrases such as “con artist” or “with artist.” I don’t consider these featured artists, but the way my code works, it’ll falsely add these artists to the featured_list
- Since some songs have more than 1 featured artist, it doesn’t make sense to cache that data into a list. A dictionary seems more appropriate here
I’m gonna work on these three errors today.
So who knows… Maybe tomorrow’s blog post will revisit this function and show how I optimized it.