Using Python to Change CSV Headers
While working on my Spotify Python project, I ran into a problem that made me shake my head. It was such a rookie mistake. I can’t “fake it till I make it” if I mess up like this all the time.
Anyway, what happened is I accidentally gave my CSV file the wrong column names.
So I had to fix it.
And earlier in the project, I used Pandas to rename columns. I copied the script, then realized not all Python Developers use Pandas every time they need to edit a CSV like this.
That made me wonder how to rename columns without using pandas.
So I went to my friend ChatGPT for help.
I learned how to edit CSV headers using Python’s built-in read/write functions.
After this, I created a make-shift CSV file with incorrect column headers. Then I wrote the following two Python scripts to clean it:
This first one uses Pandas.

This second one uses Python’s built-in read/write functions.
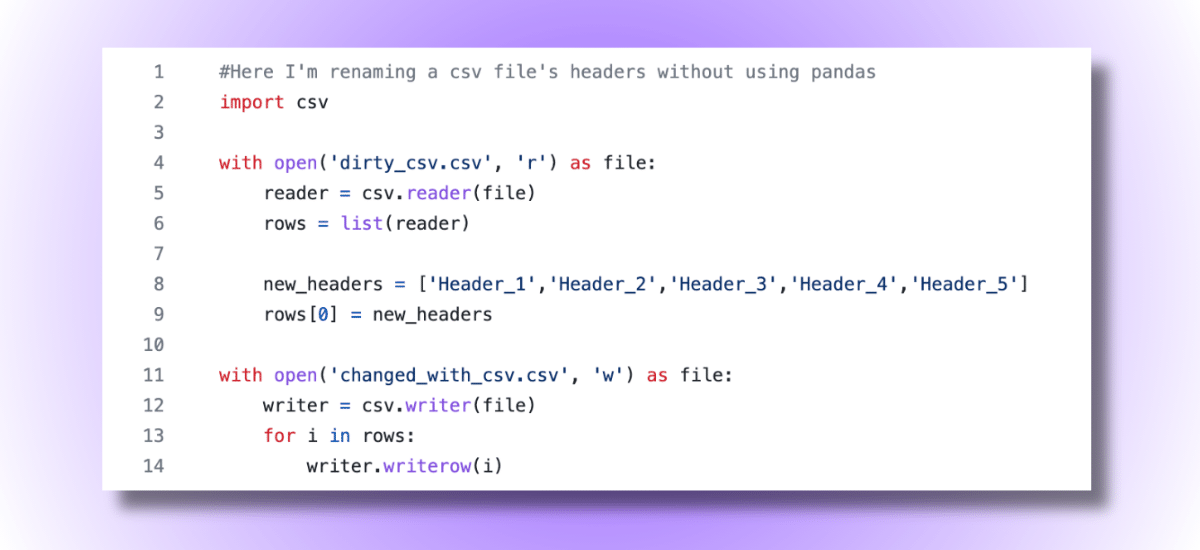
Here are two interesting observations:
- Pandas code only takes 4 lines while other code takes 10
- The Pandas looks like the more efficient option since it’s less code, but the second one is actually more effective.
The reason behind this is that the Pandas code has to read the CSV file and create an object-oriented Data Frame. This takes up a lot of time and uses a lot of memory to run. But the read/write code reads a CSV file and modifies the rows in memory so you don’t have to create the object-oriented Data Frame.
You’d expect shorter code to always be better, but this is a prime example where that’s not the case.