The Lazy (Yet Genius) Coder
Wanna hear something crazy? Wanna know how lazy I am?
I’m so lazy that I wrote a Python script so I don’t have to manually move images from my downloads folder to another folder in my Mac Finder.
But guess what…
I did it all for this experiment.
Yup, it turns out I’m designing 5x more images per blog post than expected. Since I use Canva to design images, every time I download a new one, it goes directly to my Downloads folder – as it should. But that’s annoying. I try to be organized, so I like keeping similar images in the same folder. After two days of the experiment, guess how many images I’ve created and downloaded so far…
I’ll wait…
That number is 15.
15 damn images. It’s too much. And if you add the number or images in this post too, it equals 22. So yeah, I’ve gone a little picture crazy.
But I’m learning how to freaking code right now. So I don’t have to worry about repetitive tasks. I can just automate them. And that’s what I’ve done for this experiment. I wrote a short Python script that moves all of the images I download for these blog posts and move them from my Downloads folder to my 66_dmp folder.
And btw, 66_dmp doesn’t stand for “66 dump.” It stands for 66 Days of Math and Programming.”
Anyway, here’s the code I wrote.
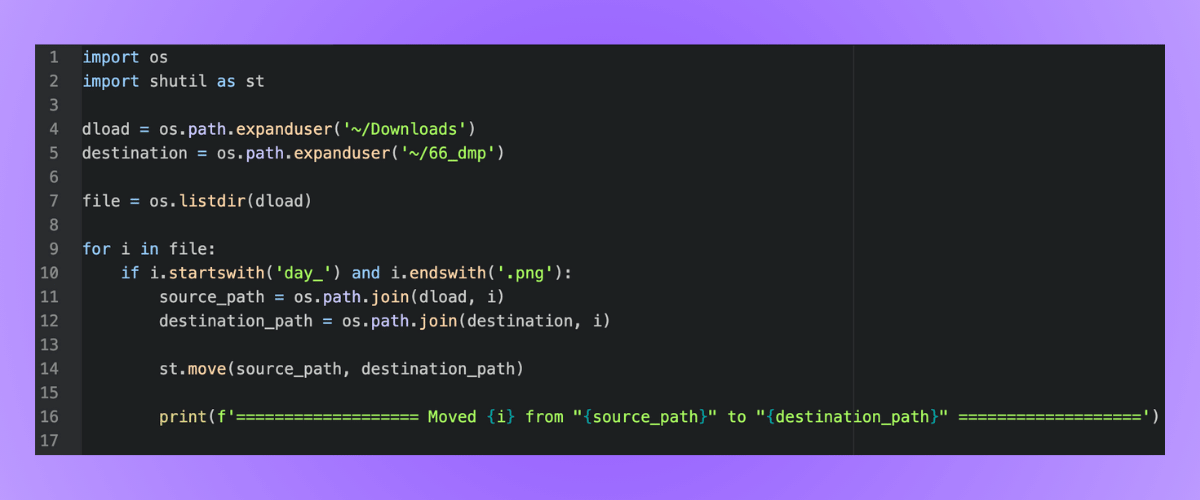
If you don’t understand all of it, don’t worry. I’ll explain what it means line by line.
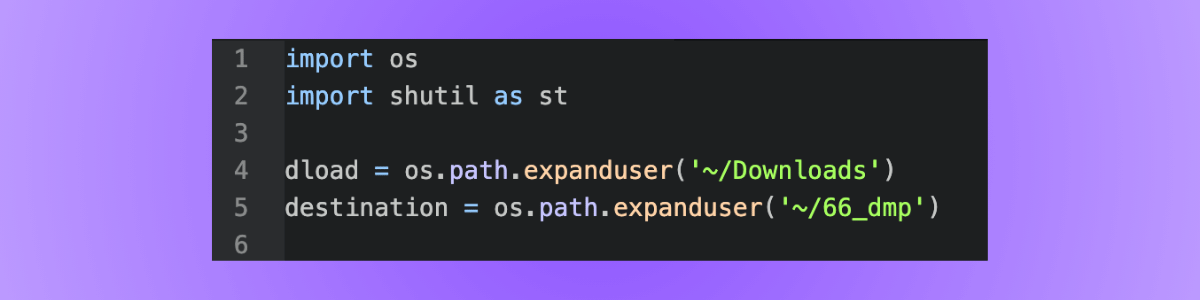
The first few lines are simple. On 1 and 2, I’m downloading the necessary libraries I need to access my Mac directory and move files around:
- Os
- Shutil
And right below that, I define two variables dload and destination. The “os.path.expanduser” uses the os library to point to a specific directory on my laptop. And the “~” used in the parameter is a placeholder for my computer’s home directory.
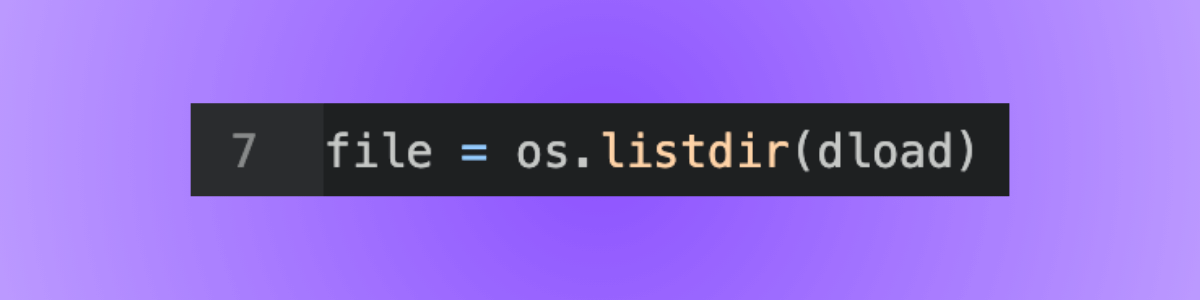
Next, I define file as “os.listdir(dload)” and that looks a bit confusing, right? Don’t worry it’s simple.
The os.listdir(dload) lists all the files and directories in the dload path I defined on line 4.

We hit the exciting part of the code on line 9.
This for loop iterates through the file I defined on line 7. And the if statement below it creates a parameter that I only care about files that start with “day_” and end with “.png.” This means that my code will look for any image in my Downloads folder whose name starts with ‘day_.”
Now look at the source_path and destination_path variables I nested in the if statement.
The “os.path.join()” function takes two paths (in this case dload/destination and the file i that passes the if statement’s parameters) and combines them into one path.

The st.move() function is the bread and butter for this code. It’s the function that actually moves images from the source path to the destination path.

Finally, I’ve got a good ole print statement. It’s not integral to the code, but it’s a cool add-on. Whenever I run the script, the print statement lets me know that the code works and the files I want to move are successfully moved.
Wrapping up
This wasn’t anything fancy or extraordinary. But its simplicity shows the beauty of Python. Even if you’re not an expert yet (like me), there are useful ways to implement programming into your everyday life.
Another cool way I’ve implemented Python into my life is by using it to collect my data from an app I used to frequently use. Check it out here.