How to Use Strptime() and Strftime() From Python's Datetime Library
While working on my email automation project, I realized that over time I want to update my MySQL database and add new items whenever I write a new blog post.
That process involves finding the most recent date in my MySQL blog_post_date column and then iterating through every item scraped from my blog to find the ones with a date greater than the greatest date from my table.
And both Python and MySQL call one date greater than another if it comes second.
So July 1st, 2023 would be greater than June 30th, 2023.
The process of finding the greatest date isn’t hard.
It’s as simple as using Python to execute the following SQL query:

But there’s a bigger issue I faced.
One that made me unnecessarily debug code for 30 minutes.
To store dates in MySQL, I need to use the DATE data type. This uses the following format: yyyy-mm-dd.
But when scraping my website, I get blog post dates as a string in this format: Month Day, Year.
I can’t add rows to my MySQL database if the date is formatted as a string.
That’s why I created this reverse_time() function.
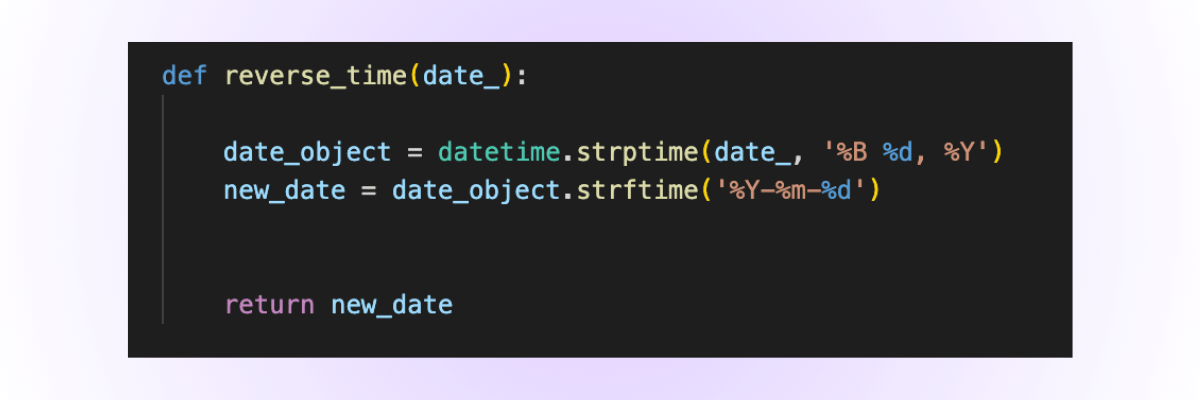
The date_ parameter is a string with the Month Day, Year format. (Ex: July 22, 2023)
Using the “datetime.strptime()” function, I turn the string into a datetime object with the same format. Then the “datetime.strftime()” function turns that datetime object into a string with the yyyy-mm-dd format MySQL likes.
Nice and simple, right?
Sadly I made a crucial mistake while creating this function. A mistake that wasted 30 minutes of my time debugging code.
So what was that mistake…
I didn’t take the time to understand how the two datetime functions worked and the data types for each function’s parameter.
You see, even though the values they return may look similar, they’re not.
Here’s the difference between what they take as parameters and the values they return…

If I had read the datetime library’s documentation, I would have known right away how to properly use the two functions.
But since I tried guessing, I wasted valuable time.
So let this be a lesson…
Don’t be greedy when you’re programming. Take the extra few minutes to read the documentation and understand the parameters/return values for the Python libraries you use.